반응형
node 설치하기
npm - n 명령어로 노드 설치
node와 npm 설치 확인
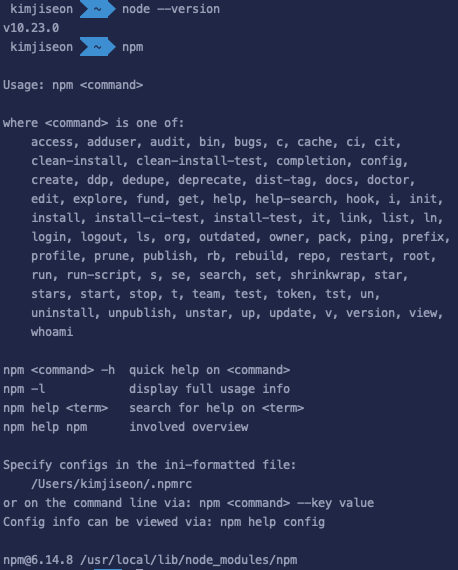
npm으로 typescript 설치하기
sudo npm install -g typescript
프로젝트 생성
- 파일 생성
touch hello.ts
- typescript를 javascript로 빌드
tsc hello.ts
- 빌드한 js 파일을 실행
node hello.js
- ts-node 명령어 깔기
npm i -g ts-node
- ts-node 명령어로 typescript 파일 실행
ts-node hello.ts
프로젝트 만들기
- 새로운 프로젝트 디렉토리를 생성 후 npm init 명령어를 통해 package.json 파일을 만든다.
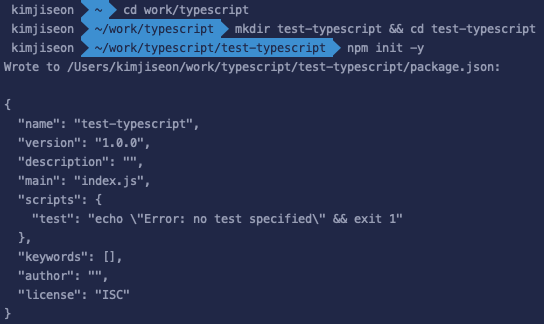
- 전역으로 ts-node 를 설치했지만 다른 개발자의 컴퓨터에는 전역으로 설치가 안 돼있을 수 있기 때문에 패키지를 -D 옵션으로 package.json 에 등록
- npm i -D typescript ts-node
- Promise 와 같은 타입을 사용하려면 @types/node 패키지 설치
- npm i -D @types/node
tsconfig.json 파일 만들기
- 타입스크립트 컴파일러의 설정 파일인 tsconfig.json 파일은 tsc --init 명령어로 생성한다.
- 열어보면 옵션들이 비활성화 되어 있음
- 다음과 같이 수정해준다.
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"outDir": "dist",
"downlevelIteration": true,
"noImplicitAny": false,
"moduleResolution": "node",
"baseUrl": ".",
"esModuleInterop": true,
"inlineSourceMap": true,
"paths": { "*":["node_modules/*"] },
},
"include": ["src/**/*"]
}
반응형
src 디렉토리와 소스파일 만들기
mkdir -p src/utils
touch src/index.ts src/utils/makePerdon.ts
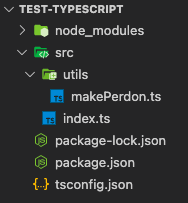
index.ts
import {testMakePerson} from './utils/makePerson'
testMakePerson()
makePerson.ts
export function makePerson(name:string, age:number) {
return {name:name, age:age}
}
export function testMakePerson() {
console.log(
makePerson('Jane',22),
makePerson('Jake',33)
)
}
package.json 수정
- 개발할 때는 ts-node를 사용하지만, 개발 완료되면 타입스크립트를 자바스크립트로 변환해서 node로 실행해야 되기 때문에 수정해줍니다.
{
"name": "test-typescript",
"version": "1.0.0",
"description": "",
"main": "src/index.js",
"scripts": {
"dev": "ts-node src",
"build": "tsc && node dist"
},
...
- dev 명령 : 개발 중에 src 디렉토리에 있는 index.ts 파일을 실행하는 용도
- build 명령 : 개발 완료 후 배포하기 위해 dist 폴더에 ES5 자바스크립트 파일을 만들 때 사용
→ 실행 명령어 : npm run dev
npm run dev, npm run build
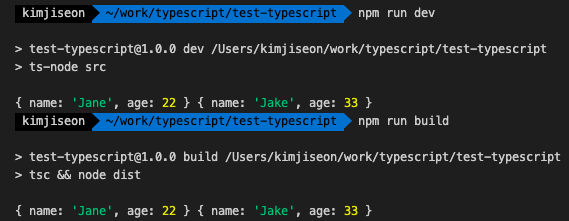
build 명령어 입력시 dist 폴더가 생깁니다.
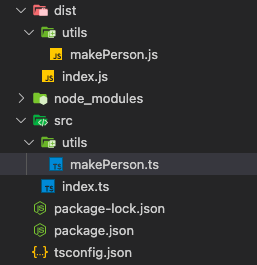
패키지 설치
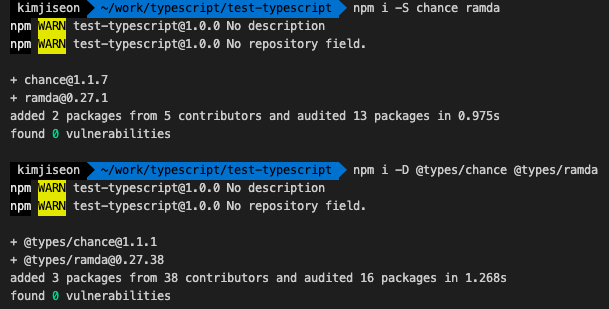
반응형